In this article I will create a Python URL Shortener without using any API. You can use my code to create your own URL Shortener.
Before starting let’s answer some questions:
Is this Python URL shortener free?
Yes, it is totally free to use.
How many links you can shortener using this code?
You can Shortener as many links as you want. You can use this code to create real time application for URL shortener. It is always free.
I will provide following two types of Python URL Shortener:
- Python URL Shortener without GUI (Graphical User Interface)
- Python URL Shortener with GUI (Graphical User Interface)
So, let’s dig in and create our URL Shortener.
In short Let’s discuss What is URL Shortener? Why should we use URL Shortener?
Introduction to URL Shortener
URL means Universal Resource Locator. Any Video, Article or Website on internet has a specific URL. If you want to share video or article you need its URL. Sometimes this URL is very long in length. URL Shortener is used to shortener any URL. It makes a long URL very short. You can easily share URL on other platforms.
Thus, in this article we will learn how to create a URL Shortener with Python. We will create a proper URL shortener. You can use this code to create a proper URL shortener application.
Prerequisites to Python URL Shortener
Please note the following points while creating Python URL Shortener:
I will use COLAB for Python Coding. COLAB is a Google online tool for Python Coding. It is also known as Google Code Laboratory.
So, let’s Write code for python URL Shortener.
Python URL Shortener without GUI
We will use pyshorteners python library to create URL shortener applications. First of all, search for Colab on Google or any other search engine or simply click on this link. It will show following screen:
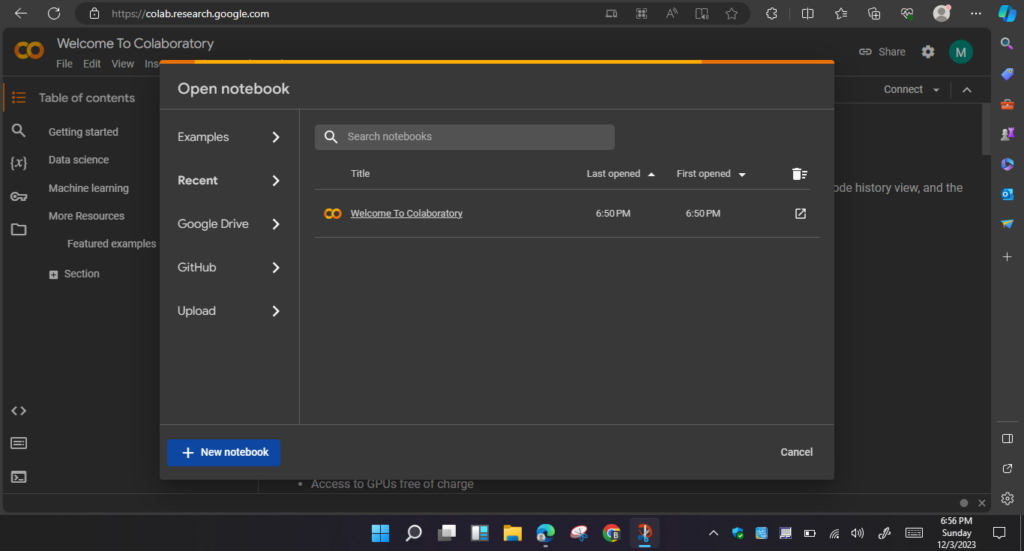
Create New Notebook Here. After creating new notebook for python. Install pyshorteners library.
!pip install pyshorteners
After successfully installing library now let’s write code for Python URL Shortener Code.
As you have installed pyshorterner library let’s import this library by writing following code:
import pyshorteners
After importing library let’s define a python function which shorteners the URL
def shorten_url(original_url):
s = pyshorteners.Shortener()
short_url = s.tinyurl.short(original_url)
return short_url
Please keep python indention rules while writing above code. The above function takes one URL as parameter and returns the URL after shortener it is using tinyurl built in function.
Now let’s create main function to run our code:
if __name__ == '__main__':
original_url = input('Enter the original URL: ')
short_url = shorten_url(original_url)
print(f'Shortened URL: {short_url}')
The above main function takes original link as input from user using prompt. Then display the new link after shortener it.
Python URL Shortener Code Output
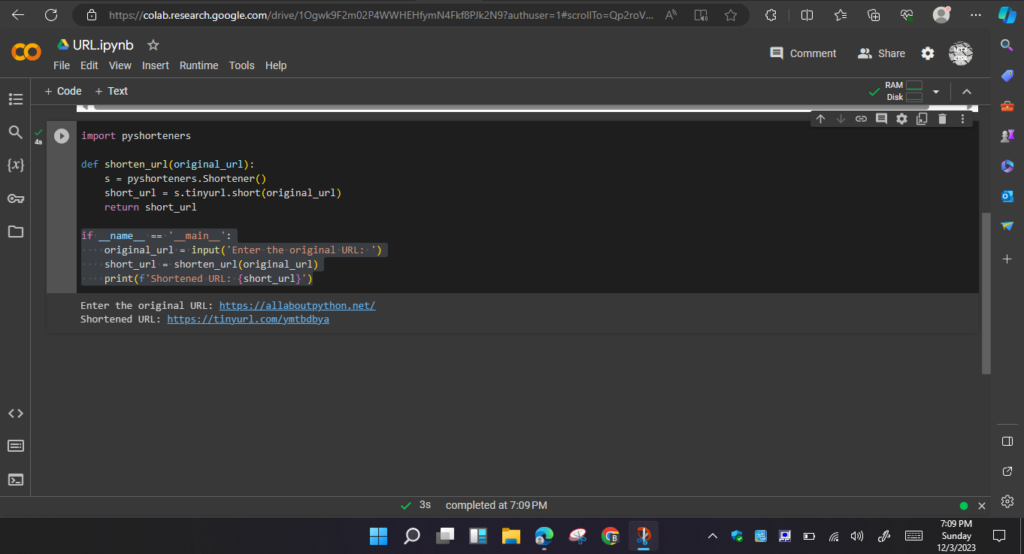
That’s all you have successfully created a proper Python URL Shortener. By using above code you can now shortener any link without paying any money to URL shortener websites.
You can view above code by using following link.
Now let’s change above code into Graphical User interface as well.
Python URL Shortener with GUI (Graphical User Interface)
To create python URL shortener with GUI I will use Python Anaconda IDE.
The first two steps are the same for this code as well. Installing library and importing library are same as above. You need to install the following library first:
!pip install tkinter
We will use tkinter library to create a GUI windows. This library will allow us to create a frame which contains buttons and boxes to take input from user.
import tkinter as tk
from tkinter import ttk
import pyshorteners
def shorten_url():
original_url = entry.get()
s = pyshorteners.Shortener()
short_url = s.tinyurl.short(original_url)
result_label.config(text=f'Shortened URL: {short_url}')
# Createing the main window
root = tk.Tk()
root.title("URL Shortener")
# Create and configure the main frame with proper padding and rows, cloumns
main_frame = ttk.Frame(root, padding="20")
main_frame.grid(column=0, row=0, sticky=(tk.W, tk.E, tk.N, tk.S))
# Create and place widgets
url_label = ttk.Label(main_frame, text="Enter the original URL:")
url_label.grid(column=0, row=0, sticky=tk.W)
entry = ttk.Entry(main_frame, width=40)
entry.grid(column=1, row=0, sticky=(tk.W, tk.E))
shorten_button = ttk.Button(main_frame, text="Shorten URL", command=shorten_url)
shorten_button.grid(column=2, row=0, sticky=tk.W)
result_label = ttk.Label(main_frame, text="")
result_label.grid(column=0, row=1, columnspan=3, sticky=tk.W)
# Below function executes the code
root.mainloop()
URL Shortener with GUI Code Output
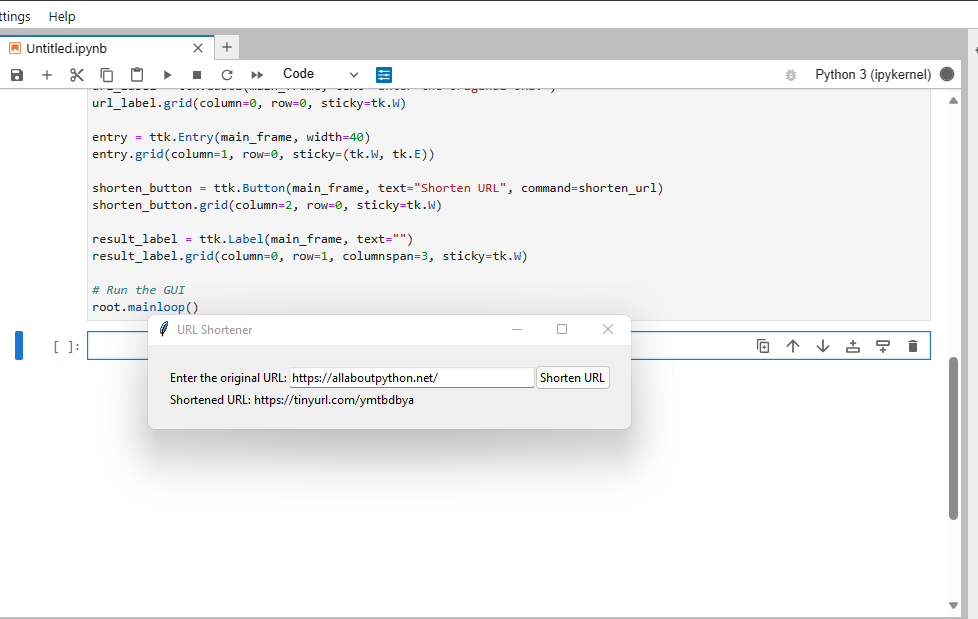
If you have any problem with installations or code, feel free to contact with me or just ask your question in comments. I’m happier to help.
I have created allaboutpython website to spread my python knowledge.