First of all, let’s discuss Control Structure in Python:
What is Control Structure in Python?
A statement used to control the flow of execution in a program is called a control structure. The instructions in a program can be organized into three kinds of control structures to control execution flow. The control structures are used to implement the program logic.
Types of Control Structures in Python
Different types of control structures are as follows:
Sequence Structure in Python
In sequential structure, the statements are executed in the same order in which they are specified in the program. The control flows from one statement to another in a logical sequence. All statements are executed exactly once. It means that no statement is skipped and no statement is executed more than once
Example: Suppose a program inputs two numbers and displays the average on screen. The program uses two statements to input numbers, one statement to calculate the average, and one statement to display the average number. These statements are executed in a sequence to find the average number. All statements are executed once when the program is executed. It is an example of a sequence control structure.
Selection Structure in Python
A selection structure selects a statement or set of statements to execute based on a condition. In this structure, a statement or set of statements is executed when a particular condition is true and ignored when the condition is false. There are different types of selection structures in Python. These are if, if…else, and switch, etc.
Example: Suppose a program inputs the marks of a student and displays a message on the screen about whether the student is pass or fail. It displays a Pass if the student gets 40 or more than 40 marks. It displays Fail when the marks are below 40. The program checks the marks before displaying the message. It is an example of a selection structure.
What is an If Statement in Python?
if Statement in Python
if is a keyword in Python language. if statement is a decision-making statement. It is the simplest form of selection constructs. It is used to execute or skip a statement or set of statements by checking a condition.
The condition is given as a relational expression. If the condition is true, the statement or set of statements after if statement is executed. If the condition is false, the statement or set of statements after if statement is not executed.
if Statement Syntax
The syntax of the if statement is as follows:
if (condition) statement;
The above syntax is used for a single statement. A set of statements can also be made conditional. The set of statements is also called compound statements.
The syntax for compound statements in if statement is as follows:
if (condition) statement 1; statement 2; statement N;
Limitation of simple if Statement in Python
if statement is the simplest selection structure but it is very limited in its use. The statement or set of statements is executed if the condition is true. But if the condition is false then nothing happens. A user may want to:
Execute one statement or set of statements if the condition is true. Execute other statement or set of statements if the condition is false.
In this situation, simple if statement cannot be used effectively.
Example
For example, a program should display ‘Pass’ if the student gets 40 or more marks. It should display ‘Fail’ if the student gets less then 40 marks. Simple if statement cannot be used to handle this situation.
If Statement Programming Examples:
Write a program in Python that inputs marks and display “Cong. You have Passed” if marks are greater than 40.
marks = int(input("Enter your marks: "))
if marks > 40:
print("Congratulations, you have passed!")
Output:
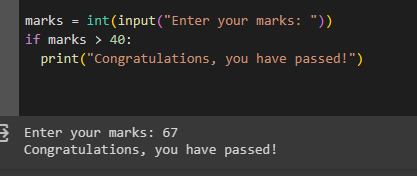
Write a program in Python that inputs two numbers and finds whether both numbers are equal?
# prompt: write a program that inputs two numbers and finds whether both numbers are equal?
x = int(input("Enter first number: "))
y = int(input("Enter second number: "))
if x == y:
print("Both numbers are equal")
Output:
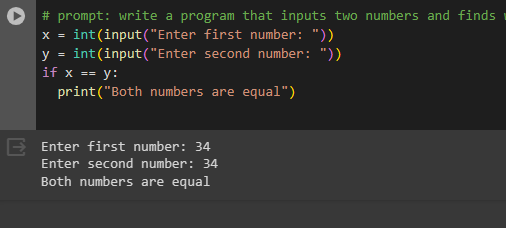
write a python program that inputs two numbers and finds whether the second number is the square of the first.
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
if num2 == num1**2:
print("Yes, second number is square of first number")
Output:
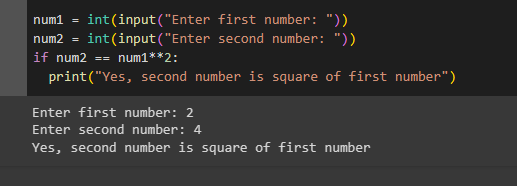
write a python program that inputs marks of three subjects. if the average mark is more than 80, it should display two messages “You are above standard!” and “Your admission is granted”.
marks1 = float(input("Enter marks of subject 1:"))
marks2 = float(input("Enter marks of subject 2:"))
marks3 = float(input("Enter marks of subject 3:"))
average = (marks1 + marks2 + marks3) / 3
if average > 80:
print("You are above standard!")
print("Your admission is granted")
Output:
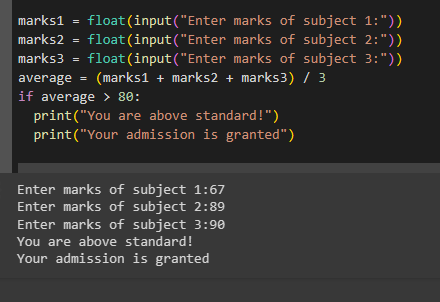
write a Python program that inputs marks of three numbers and displays the greatest number using an if statement only.
a=int(input("enter the first number:"))
b=int(input("enter the second number:"))
c=int(input("enter the third number:"))
max = a
if b>max:
max=b
if c>max:
max=c
print("Greatest Number = ", max)
Output:

Keep in Mind indention. You have to put five spaces or a tab after the if condition in Python.
Also, check Python Projects.